Introduction
filament allows for a data driven way to add items, blocks and decorations/furniture to your farbic-based minecraft server.
Checkout usage or "creating content" or the example datapack in the GitHub repo to get started!
There is also a test server that you can join with 1.20.5-1.21 clients to get a gimple of what is possible at mc.tomalbrc.de, default minecraft java port
Using filament
Requires fabric api and polymer by patbox
filament mainly loads additional content using datapacks;
It also loads any assets from the assets
folder in datapacks, if present.
The datapack structure should look something like this:
data/
|-- <namespace>/
| |-- filament/
| | |-- item/
| | | |-- <item_config>.json
| | | |-- <item_config>.yaml
| | |
| | |-- block/
| | | |-- <block_config>.json
| | | |-- <block_config>.yaml
| | |
| | |-- decoration/
| | | |-- <decoration_configs>.json
| | |
| | |-- model/
| | |-- <blockbench_model>.bbmodel
| | |-- <animated-java_model>.ajmodel
|
assets/
| | ...
pack.mcmeta
So a configuration file path for a new block could look like this:
MyDatapack/data/<namespace>/filament/block/myblock.json
The files can also be located in subfolders for better organization:
MyDatapack/data/<namespace>/filament/block/stone_like/myblock.json
Filament supports yaml files as of 0.12.0.
Blockbench and Animated-Java models for decorations are supported using the blockbench import library
In versions 1.21.4 and higher, filament will automatically generate the item asset json under namespace/items/itemname.json
.
You can provide your own item asset models using the itemModel
field of an item (in the root structure of an item config) or by providing an item_model components using the components
field.
Common fields
Item-, block- and decoration configuration files share some common fields.
Note: All fields support either camelCase or snake_case!
Those are:
id
: Identifier of the item/block/decoration.vanillaItem
: The client-side item to use. This will be used for custom model data values in <= 1.21.1blockResource
: Here you can specify models for different blocks-states or just a single model for simple blocks.itemResource
: Specifies the model of the item. Some item-behaviours may require you to provide models for their "states" (i.e. thetrap
behaviour)properties
: Properties differ for each config type, but all share the item properties.
Behaviours
Custom content is driven by something called behaviours
in filament.
Item behaviours give items, block-items, blocks and **decorations as well as decoration-items special capabilities, not normally possible using just vanilla item components.
This means that items, blocks and decorations are all capable of all item behaviours.
Block behaviours are not compatible with decorations and vice versa.
Genuinely modded content!
On the server-side of things, all your custom items and blocks are genuinely modded, meaning they have their own id, instead of 'just' being vanilla items with components, only obtainable via recipes for example.
You can for example just use /give @s mynamespace:myitem
instead of having to provide a dozen components or a datapack function.
For clients that don't have any mods or polymer installed, the item show up as the vanillaItem
in the config for your content, but with all components correctly applied with your custom models.
In 1.21.1, custom model data override predicates will be automatically generated. You can also set the vanilla custom model data components in the components
field of a config to use your own values.
This is not necessary in version 1.21.2 and above.
For 1.21.4, the new item model will be automatically generated. You can set the itemModel
to prevent that.
Third party compatibility
Filament has 2 options to make
- Decoration
blocks
andseat
rotations (seat
behaviour) more similar to that of Oraxen / ItemsAdder - filaments placement for those is rotated by 180° b default. - Change the display of cosmetics on the player, this uses the "head" as display context of the item. It also moves the backpack up, since filament uses item display entities instead of armor stands.
Those 2 options in filament.json are:
alternative_block_placement
alternative_cosmetic_placement
While filament does not support reading YAML files of third party mods/plugins, it should be possible to write a conversion script.
For Developers
Filament provides an API and registry for custom item, block and decoration behaviours.
Behaviours can inherit ItemBehaviour
, BlockBehaviour
and DecorationBehaviour
.
Custom behaviours can be registered using the BehaviourRegistry
.
When referencing a custom behaviour in a config file you will have to specify the whole id of the behaviour, by default filament parses behaviours without namespace in the filament namespace.
You can check out TSA: Decorations for an example Decoration Behaviour implementation or one of the various built-in behaviours.
Example for the 'instrument' item-behaviour
public class Instrument implements ItemBehaviour<Instrument.Config> {
private final Config config;
public Instrument(Config config) {
this.config = config;
}
@Override
@NotNull
public Instrument.Config getConfig() {
return this.config;
}
@Override
public InteractionResult use(Item item, Level level, Player player, InteractionHand interactionHand) {
ItemStack itemStack = player.getItemInHand(interactionHand);
player.startUsingItem(interactionHand);
play(level, player, config);
if (config.useDuration > 0) player.getCooldowns().addCooldown(itemStack, config.useDuration);
player.awardStat(Stats.ITEM_USED.get(item));
return InteractionResult.CONSUME;
}
private static void play(Level level, Player player, Config instrument) {
float f = instrument.range / 25.0F;
level.playSound(null, player, SoundEvent.createVariableRangeEvent(instrument.sound), SoundSource.RECORDS, f, 1.0F);
level.gameEvent(GameEvent.INSTRUMENT_PLAY, player.position(), GameEvent.Context.of(player));
}
public static class Config {
public ResourceLocation sound = null;
public int range = 0;
public int useDuration = 0;
}
}
Registering it
public static final BehaviourType<Instrument, Instrument.Config> INSTRUMENT = registerBehaviour("instrument", Instrument.class);
private static <T extends Behaviour<E>,E> BehaviourType<T, E> registerBehaviour(String name, Class<T> type) {
return BehaviourRegistry.registerBehaviour(ResourceLocation.fromNamespaceAndPath(MOD_ID, name), type);
}
Filament also provides methods to load config files and blockbench models from mods using mod-ids.
This is needed in case you want your datapack to work client-side without the player having reload resources, since resources are loaded with datapacks when loading a world, and clients load the assets in earlier than that.
Blockbench models require an additional identifier for the model which are used to reference it in the decoration config files later on
public class MyMod implements ModInitializer {
@Override
public void onInitialize() {
FilamentLoader.loadModels("my-modid", "mynamespace");
FilamentLoader.loadItems("my-modid");
FilamentLoader.loadBlocks("my-modid");
FilamentLoader.loadDecorations("my-modid");
PolymerResourcePackUtils.addModAssets("my-modid");
PolymerResourcePackUtils.markAsRequired();
}
}
Creating content
There are 3 different configuration formats, depending on what you want to add; Items, Blocks or Decorations.
Items:
The Items category contains items such as food, instruments, hats, armour, weapons/tools, etc.
Blocks:
Blocks with a custom texture, ranges from simple blocks, to slabs, doors, trapdoors, crops, saplings, ores, and more.
Decorations:
Blocks that rotate by 45° with multi-block, waterlogging support, seats, showcases and more! For everything that a normal block would not suffice for.
Item Groups
You can add custom item-groups for your items/blocks and decorations.
This can be done by adding an item-groups.json
file in the root of the filament
directory of your datapack.
Here is an example of an item-groups.json
file:
[
{
"id": "mynamespace:mygroup",
"item": "mynamespace:myitem"
},
{
"id": "mynamespace:myblockgroup",
"item": "mynamespace:myitem",
"literal": "<c:red>My Block Group"
}
]
Fields
id
The identifier of your item group. This is used in the individual item/block/decoration files in the field group
.
Each config. type supports the group
field.
The id is also used for the translation key, see below.
item
The id of the item shown as the Creative Tab Icon.
literal
The name displayed for the item group - supports Placeholder API's basic text formatting. By default, a translatable string is used for resource-packs, using the id
of the item-group.
The translation key looks like this: mynamespace.itemGroup.mygroup
for an id
of mynamespace:mygroup
.
So an en_US.json
for a resrouce-pack might look like this:
{
"mynamespace.itemGroup.mygroup": "My Group"
}
or for german (de_DE.json
):
{
"mynamespace.itemGroup.mygroup": "Meine Gruppe"
}
Items
File Location
Item configuration files should be placed in the following directory:
MyDatapack/data/<namespace>/filament/item/myitem.json
Example
Here is a basic example of an item configuration:
{
"id": "mynamespace:clown_horn",
"vanillaItem": "minecraft:paper",
"itemModel": "minecraft:mymodel", // optional and only available in >= 1.21.4
"itemResource": {
"models": {
"default": "mynamespace:custom/misc/clown_horn"
}
},
"properties": {
...
},
"behaviour": {
...
},
"components": {
...
}
}
Item configurations have two required fields: id
and vanillaItem
.
id
Your custom ID in the format namespace:item_name
vanillaItem
The vanilla item to "overwrite". Filament (through Polymer) will create custom_model_data
IDs for the generated resource pack. Your custom item will not inherit any other properties server-side from the vanilla item other than appearance, if the itemResource
field is not set. For interaction purposes on the client, it is important to choose the appropriate vanilla item to use here.
Optional Fields
The fields itemModel
, itemResource
, properties
, group
, and behaviour
are optional.
itemModel
Path to an item model definition (in assets/namespace/items/<name>.json
)
This overwrites the itemResource field.
itemResource
Specifies the resource(s) for the item model. Depending on the item's behaviour(s), it may use additional keys/fields in itemResource
.
Fields:
models
: An object containing model definitions.default
: The default model for the item.- Additional keys may be required depending on the item's behaviour (e.g.,
trapped
for a trap behaviour).
textures
(upcoming in future versions): An object containing texture definitions.default
: The default texture for the item.- Additional keys may be required depending on the item's behaviour.
properties
Defines various properties for the item. The structure and contents of this field will depend on the specific properties being set.
group
Defines the item-group for this item. See Item Groups for more information.
behaviour
Defines specific behaviours or interactions for the item. The structure and contents of this field will depend on the specific behaviours being set.
See Item Behaviours for more information.
components
Defines a set of vanilla minecraft components like minecraft:food
, minecraft:tool
, etc.
The format is the same that is used in datapacks. This is only supported in minecraft version 1.20.5 and later
Properties
Item properties are shared between all content types: Items, blocks and decorations. All properties are optional.
Example with all properties set:
{
"id": "mynamespace:clown_horn",
"vanillaItem": "minecraft:paper",
"models": {
"default": "mynamespace:custom/misc/clown_horn"
},
"properties": {
"durability": 100,
"stackSize": 64,
"lore": ["line 1", "line 2"],
"fireResistant": false
}
}
durability
:
The items' vanillaItem
will have to be a vanilla item that has a durability bar by default, otherwise it will not show up on the client side.
stackSize
:
Number of items a stack can hold. Should be between 1 and 99 Values above 99 or 0 and below might cause problems™
Defaults to 64
lore
:
List of strings to use as item lore. Supports PlaceholderAPI's simple text formatting.
Example: <c:red>MyLore</c>
Example: <lang:'mymod.lore.mylore'>
fireResistant
:
Boolean (true/false) wether the item is fire-resistant.
Defaults to false
Item Behaviours
Item behaviours define specific functionalities associated with items, blocks, and decorations.
All behaviours are optional, and some are mutually exclusive (e.g., trap, shoot, and instrument).
Example with some behaviours set:
Click to expand
{
"id": "mynamespace:multi_example",
"vanillaItem": "minecraft:paper",
"itemResource": {
"models": {
"default": "mynamespace:custom/misc/clown_horn",
"trapped": "mynamespace:custom/misc/clown_horn_trapped"
}
},
"behaviour": {
"instrument": {
"sound": "mynamespace:misc.honk",
"range": 64,
"useDuration": 60
},
"shoot": {
"consumes": false,
"baseDamage": 2.0,
"speed": 1.0,
"projectile": "minecraft:iron_axe",
"sound": "mynamespace:misc.shoot"
},
"armor": {
"slot": "head",
"texture": "mynamespace:texture_name"
},
"trap": {
"types": ["minecraft:villager", "minecraft:zombie", "minecraft:skeleton"],
"requiredEffects": ["minecraft:glowing"],
"chance": 75,
"useDuration": 0
},
"fuel": {
"value": 10
},
"food": {
"hunger": 2,
"saturation": 1.0,
"canAlwaysEat": true,
"fastfood": true
},
"cosmetic": {
"slot": "head",
"model": "mynamespace:custom/models/clown_backpack_animated",
"autoplay": "idle",
"scale": [1.5, 1.5, 1.5],
"translation": [0.0, 0.5, 0.0]
},
"execute": {
"consumes": true,
"command": "/summon minecraft:creeper ~ ~ ~ {powered:1b}",
"sound": "minecraft:block.anvil.place"
}
}
}
armor
behaviour
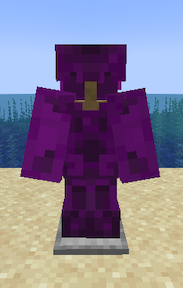
Defines armour item behaviours, using the Fancypants shader via Polymer.
As of filament 0.7 it is possible to use armor trims to render custom armor, to enable this, set the trim
flag to true
.
For Shader-Based Armor:
- The textures for the shader version of armor should be located in
assets/minecraft/textures/models/armor/
. - For a
texture
field value ofmynamespace:amethyst
, the textures should be namedamethyst_layer_1.png
andamethyst_layer_2.png
. - It's required to use a leather armor item for your
vanillaItem
for the shader to work.
For Trim-Based Armor:
- When using Armor Trims for the armor, the textures need to be located in
assets/minecraft/textures/trims/models/armor/
. - For a
texture
field value ofmynamespace:amethyst
, the textures should be namedamethyst.png
andamethyst_leggings.png
. - It's required to use an armor item for your
vanillaItem
. Any armor item should work. - Depending on the
vanillaItem
of your custom item, you might be able to see parts of the original armors texture, to mitigate this, you will have to enable thetrimArmorReplaceChainmail
option in the mods configs. - Enabling
trimArmorReplaceChainmail
will prevent all chainmail armor pieces unable to receive or display armor trims. The Smithing Table will also reject chainmail armor with this option enabled.
Fields:
slot
: The equipment slot for the armour piece (e.g., head, chest, legs, or feet).texture
: The resource location of the texture associated with the armour. Example:mynamespace:amethyst
trim
: Flag whether to use trim-based armor instead of shaders
compostable
behaviour

Makes the item usable in composters.
Fields:
chance
: Chance of raising the composter level by 1 between 0 and 100villagerInteraction
: Allows farmer villagers to compost the item. Defaults totrue
cosmetic
behaviour
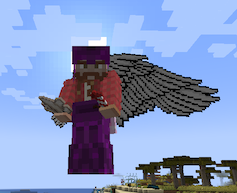
Defines cosmetic item behaviour for either the head or chestplate slot, supporting both Blockbench models for chestplates and simple item models for either slot.
Fields:
slot
: The equipment slot for the cosmetic (head or chest).model
: Optional, the resource location of the animated blockbench or animated-java model for the cosmetic.autoplay
: Optional, the name of the animation to autoplay, which should be loopable.scale
: Scale of the chest cosmetic, defaulting to (1, 1, 1).translation
: Translation of the chest cosmetic, defaulting to (0, 0, 0).
execute
behaviour
Executes a command on item use with the player as source, located at the player.
Fields:
consumes
: Indicates whether the execution consumes the item. Defaults tofalse
command
: The command string to execute. Empty by defaultsound
: Optional sound effect to play during execution. Empty by default
food
behaviour

Defines food item behaviour for edible items.
Fields:
hunger
: The amount of hunger restored when consumed. Defaults to1
saturation
: The saturation modifier provided by the food. Defaults to0.6
canAlwaysEat
: Indicates whether the item can be eaten when the hunger bar is full. Defaults tofalse
fastfood
: Boolean indicating whether the food item is considered fast food (eats faster than normal). Defaults tofalse
villager_food
behaviour

Makes the item edible for villagers (for villager breeding).
Fields:
value
: The amount of "breeding power" the item has (1 = normal food item, 4 = bread). Defaults to1
fuel
behaviour
Defines fuel behaviour for items, specifying their value used in furnaces and similar item-burning blocks.
- Fields:
value
: The value associated with the fuel, determining burn duration. Defaults to10
instrument
behaviour

Defines instrument behaviour for items, similar to goat horns.
- Fields:
sound
: The sound associated with the instrument. Empty by defaultrange
: The range of the instrument. Defaults to0
useDuration
: Delay in ticks for using the instrument. Defaults to0
stripper
behaviour
Gives the item the ability to strip Logs/scrape copper blocks, like an axe. Uses 1 durability.
trap
behaviour

Defines trap behaviour for items capable of trapping specific entity types.
- Fields:
types
: List of allowed entity types to trap. Example:["minecraft:silverfish", "minecraft:spider"]
requiredEffects
: List of required effects for the trap. Example:["minecraft:weakness"]
chance
: Chance of the trap triggering (0
-100
). Defaults to50
useDuration
: Use cooldown for the trap item. Defaults to0
banner_pattern
behaviour
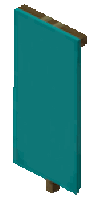
Allows you to assign a banner pattern to an item for use in Looms.
See the mynamespace:bannertestitem
item config in the example datapack in the GitHub repo.
- Fields:
id
: The id of your banner_pattern in your datapack. Empty by default
bow
behaviour

Vanilla-like bow behaviour. Lets you specify which item can be shot, but anything that is not an arrow or firework rocket will render as normal arrow. Allows to specify a power multiplier for shooting power. Supports firework rockets.
Make sure to use minecraft:bow
as vanillaItem
in order for the item model overrides to work properly!
- Fields:
powerMultiplier
: The power multiplier. Defaults to3
supportedProjectiles
: List of supported items in the inventory for use with the bow. Defaults to["minecraft:arrow", "minecraft:spectral_arrow"]
supportedHeldProjectiles
: List of supported items for use when in main/offhand. Defaults to["minecraft:arrow", "minecraft:spectral_arrow", "minecraft:firework_rocket"]
shootSound
: The sound when shooting a projectile. Default tominecraft:entity.arrow.shoot
This behaviour can automatically generate the item model predicate overrides for bows (item assets in `items` in 1.21.4).
In order to automatically generate an item model for bows, you have to provide models for default
, pulling_0
, pulling_1
and pulling_2
in the itemResource
field:
{
"itemResource": {
"models": {
"default": "minecraft:custom/bow/custombow",
"pulling_0": "minecraft:custom/bow/custombow_pulling_0",
"pulling_1": "minecraft:custom/bow/custombow_pulling_1",
"pulling_2": "minecraft:custom/bow/custombow_pulling_2"
}
}
}
crossbow
behaviour

Vanilla-like crossbow behaviour. Lets you specify which item can be shot, but anything that is not an arrow or firework rocket will render as normal arrow. Allows to specify a power multiplier for shooting power.
Make sure to use minecraft:crossbow
as vanillaItem
in order for the item model overrides to work properly!
This behaviour can automatically generate the item model predicate overrides for crossbows (item assets in items
in 1.21.4).
In order to automatically generate an item model for crossbows, you have to provide models for default
, pulling_0
, pulling_1
, pulling_2
, arrow
and rocket
in the itemResource
field:
{
"itemResource": {
"models": {
"default": "minecraft:custom/crossbow/crossy1", // model without projectile
"pulling_0": "minecraft:custom/crossbow/crossy1_pulling_0",
"pulling_1": "minecraft:custom/crossbow/crossy1_pulling_1",
"pulling_2": "minecraft:custom/crossbow/crossy1_pulling_2",
"arrow": "minecraft:custom/crossbow/crossy1_arrow", // model with projectile
"rocket": "minecraft:custom/crossbow/crossy1_rocket" // model with projectile
}
}
}
Fields:
powerMultiplier
: The power multiplier. Defaults to1
supportedProjectiles
: List of supported items in the inventory for use with the crossbow. Defaults to["minecraft:arrow", "minecraft:spectral_arrow"]
supportedHeldProjectiles
: List of supported items for use when in main/offhand. Defaults to["minecraft:arrow", "minecraft:spectral_arrow", "minecraft:firework_rocket"]
shootSound
: The sound when shooting a projectile. Default tominecraft:item.crossbow.shoot
loadingStartSound
: Projectile loading start sound. Default tominecraft:item.crossbow.loading_start
loadingMiddleSound
: Projectile loading middle sound. Default tominecraft:item.crossbow.loading_middle
loadingEndSound
: Projectile loading end sound. Default tominecraft:item.crossbow.loading_end
shoot
behaviour
Defines behaviour for items capable of shooting custom projectiles or being shot themselves.
Fields:
consumes
: Indicates whether shooting consumes the item. Defaults tofalse
baseDamage
: The base damage of the projectile. Defaults to2.0
speed
: The speed at which the projectile is fired. Defaults to1.0
projectile
: The identifier for the projectile item. Empty by defaultsound
: Optional sound effect to play when shooting. Empty by defaulttranslation
: Translation offset for the projectile. Defaults to[0 0 0]
rotation
: Rotation for the projectile. Defaults to[0 90 0]
scale
: Scale for the projectile. Defaults to0.6
shield
behaviour
Makes the item usable as shield.
This behaviour can automatically generate the item model predicate overrides for shields (item assets in items
in 1.21.4).
In order to automatically generate an item model for shields, you have to provide models for default
and blocking
in the itemResource
field:
{
"itemResource": {
"models": {
"default": "minecraft:custom/shield/shield1",
"blocking": "minecraft:custom/shield/shield1_blocking"
}
}
}
fishing_rod
behaviour
Makes the item behave like a fishing rod!
This behaviour can automatically generate the item model predicate overrides for fishing rods (item assets in items
in 1.21.4).
In order to automatically generate an item model for fishing rods, you have to provide models for default
and cast
in the itemResource
field:
{
"itemResource": {
"models": {
"default": "minecraft:custom/rod/fire_rod",
"cast": "minecraft:custom/rod/fire_rod_cast"
}
}
}
enchantable
behaviour
Backport of the minecraft:enchantable
component introduced in 1.21.2, only available for 1.21.1.
Fields:
value
: Enchantability value. Defaults to1
Example:
{
"behaviour": {
"enchantable": {
"value": 1
}
}
}
Examples
See the datapack in the GitHub repository for more examples!
https://github.com/tomalbrc/filament/tree/main/example_datapack
Note: All fields support either camelCase or snake_case!
Clown horn intrument:
JSON:
{
"id": "mynamespace:clown_horn",
"vanillaItem": "minecraft:paper",
"itemResource": {
"models": {
"default": "mynamespace:custom/misc/clown_horn"
}
},
"properties": {
"stackSize": 1
},
"behaviour": {
"instrument": {
"sound": "mynamespace:misc.honk",
"range": 64,
"useDuration": 60
}
}
}
YAML:
id: mynamespace:clown_horn
vanilla_item: minecraft:paper
item_resource:
models:
default: mynamespace:custom/misc/clown_horn
properties:
stack_size: 1
behaviour:
instrument:
sound: mynamespace:misc.honk
range: 64
use_duration: 60
Allay trap:
JSON:
{
"id": "mynamespace:allay_bottle",
"vanillaItem": "minecraft:carrot_on_a_stick",
"itemResource": {
"models": {
"default": "mynamespace:custom/traps/allay_bottle",
"trapped": "mynamespace:custom/traps/allay_bottle_trapped"
}
},
"properties": {
"durability": 20,
"stackSize": 1
},
"behaviour": {
"trap": {
"types": ["minecraft:allay"],
"useDuration": 120
}
}
}
YAML:
id: mynamespace:allay_bottle
vanilla_item: minecraft:carrot_on_a_stick
item_resource:
models:
default: mynamespace:custom/traps/allay_bottle
trapped: mynamespace:custom/traps/allay_bottle_trapped
properties:
durability: 20
stack_size: 1
behaviour:
trap:
types:
- minecraft:allay
use_duration: 120
Hat (can be put into inventory/swapped like normal helmets)
JSON:
{
"id": "mynamespace:magic_hat",
"vanillaItem": "minecraft:paper",
"itemResource": {
"models": {
"default": "minecraft:custom/hats/magic_hat"
}
},
"properties": {
"stackSize": 1
},
"components": {
"minecraft:equippable": {
"slot": "head",
"swappable": true,
"damage_on_hurt": true,
"equip_on_interact": false
}
}
}
YAML:
id: mynamespace:magic_hat
vanilla_item: minecraft:paper
item_resource:
models:
default: minecraft:custom/hats/magic_hat
properties:
stack_size: 1
components:
minecraft:equippable:
slot: head
swappable: true
damage_on_hurt: true
equip_on_interact: false
Blocks
File
Block configuration files are to be placed in MyDatapack/data/<namespace>/filament/block/myblock.json
Most Item behaviours such as food
, fuel
, cosmetic
and more are supported by blocks.
You can also set components for the block-item, similar to item configurations using the components
field
Contents
Blocks like candles or turtle eggs, with mutiple blocks in 1:
{
"id": "mynamespace:pebbles",
"vanillaItem": "minecraft:paper",
"blockResource": {
"models": {
"count=1": "mynamespace:custom/block/stone/pebbles_1",
"count=2": "mynamespace:custom/block/stone/pebbles_2",
"count=3": "mynamespace:custom/block/stone/pebbles_3",
"count=4": "mynamespace:custom/block/stone/pebbles_4"
}
},
"itemResource": {
"models": {
"default": "mynamespace:custom/block/stone/pebbles_item"
}
},
"blockModelType": "biome_plant_block",
"properties": {
"destroyTime": 0,
"blockBase": "minecraft:stone"
},
"group": "mynamespace:myblockgroup",
"components": {
...
}
}
The fields id
, blockResource
, and blockModelType
are required to be set.
Fields:
id
(required):
Identifier of the block and its item.
Example: mynamespace:myblock
vanillaItem
:
The vanilla item that is sent to the client and gets skinned using CustomModelData internally.
Defaults to minecraft:paper
blockResource
(required):
An object that allows you to provide different block models for each block-state-property that may be provided by a block behaviour.
For now, it is only possible to provide block models directly, support for just textures is planned for a future version.
The keys work similar to the vanilla blockstate files in resourcepacks, you specify the model to use based on the block-state.
An example for the count
block-behaviour:
{
"blockResource": {
"models": {
"count=1": "mynamespace:custom/block/stone/pebbles_1",
"count=2": "mynamespace:custom/block/stone/pebbles_2",
"count=3": "mynamespace:custom/block/stone/pebbles_3",
"count=4": "mynamespace:custom/block/stone/pebbles_4"
}
}
}
itemModel
Path to an item model definition (in assets/namespace/items/<name>.json
)
This overwrites the itemResource field.
itemResource
:
An object that allows you to provide different item-models which may be required by some item-behaviours.
The trapped
behaviour for example requires a model for the trapped
key.
Example
{
...,
"itemResource": {
"models": {
"default": "mynamespace:custom/trap/allay_trap"
"trapped": "mynamespace:custom/trap/allay_trap_filled"
}
},
...
}
blockModelType
(required in most cases):
The block model to use/retexture. See Block Model Types for a list of options.
For some block behaviours like slab
it may be required to leave this field empty!
properties
:
The properties of this block. See Block Properties for details.
group
Defines the item-group for this blocks' item. See Item Groups for more information.
components
:
An object with minecraft components used for the item.
Example:
{
...
"components": {
"minecraft:tool": {
"default_mining_speed":1.0,
"damage_per_block":2,
"rules":[
{
"speed":15,
"correct_for_drops":true,
"blocks":"#sword_efficient"
},
{
"speed":1.5,
"blocks":"cobweb"
}
]
}
},
...
}
Block model types
blockModelType
values are available through polymer and limited in number, since they re-use block-states that are visually the same on the client.
The following options are availble, with their amount:
blockModelType | Amount of blocks |
---|---|
full_block | 1149 |
transparent_block | 52 |
transparent_block_waterlogged | 52 |
biome_transparent_block | 78 |
biome_transparent_block_waterlogged | 65 |
farmland_block | 5 |
vines_block | 100 |
plant_block | 7 |
biome_plant_block | 15 |
kelp_block | 25 |
cactus_block | 15 |
tripwire_block | 32 |
tripwire_block_flat | 32 |
top_slab | 5 |
top_slab_waterlogged | 5 |
bottom_slab | 5 |
bottom_slab_waterlogged | 5 |
See the polymer documentation for more infos about the properties of the block model types
When choosing blocks that break instantly on the client, like plant_block or tripwire_block for example, the destroyTime property in the block config has to be 0 as well.
You can map the blockModelType
field of block configs to blockstates, this allows you to change the hitbox of the block depending on the block-state.
In some cases, for example when using the simple_waterloggable
behaviour, you might want to specify the waterlogged state for your custom block.
Example:
{
"id": "mynamespace:half_slab",
"blockResource": {
"models" : {
"waterlogged=false": "minecraft:custom/block/dirt/dirt_slab",
"waterlogged=true": "minecraft:custom/block/dirt/dirt_slab"
}
},
"itemResource": {
"models" : {
"default": "minecraft:custom/block/dirt/dirt_slab"
}
},
"behaviour": {
"simple_waterloggable": {}
},
"blockModelType": {
"waterlogged=false": "sculk_sensor_block",
"waterlogged=true": "sculk_sensor_block_waterlogged"
},
"properties": {
"blockBase": "minecraft:dirt"
}
}
Block Properties
Block properties share the same properties as items
All properties (excluding the shared item and decoration properties):
{
"properties": {
"blockBase": "minecraft:stone",
"itemBase": "minecraft:paper",
"requiresTool": true,
"explosionResistance": 10,
"destroyTime": 5,
"redstoneConductor": false,
"lightEmission": 0
}
}
blockBase
:
Specifies the base block for this block property. Must be a valid block identifier. This is used for sounds and particles.
Defaults to minecraft:stone
requiresTool
:
Boolean (true/false) indicating whether the block requires a specific tool to be harvested.
Defaults to true
explosionResistance
:
Number indicating the block's resistance to explosions.
Defaults to 0
destroyTime
:
Resistance of the block/the time required to destroy the block.
The destroyTime is used as explosionResistance if explosionResistance is not explicitly specified.
For indestructible blocks use a destroyTime of -1.
Defaults to 0
redstoneConductor
:
Boolean (true/false) indicating whether the block can conduct redstone signals.
The value of this property can be mapped to a blockstate like this:
{
"properties": {
"redstoneConductor": {
"powerlevel=0": true,
"powerlevel=1": false,
...
}
}
}
Defaults to true
lightEmission
:
Light level this block emits.
The value of this property can be mapped to a blockstate like this:
{
"properties": {
"lightEmission": {
"powerlevel=0": 0,
"powerlevel=1": 1,
...
}
}
}
Defaults to 0
transparent
:
Flag indicating whether the block is transparent. Transparent blocks don't block light
Defaults to false
allowsSpawning
:
Flag indicating whether mobs can spawn on this block.
Defaults to false
replaceable
:
Flag indicating whether this block can be replaced by another block when placing a new block (e.g., grass can be replaced when placing a solid block).
Defaults to false
collision
:
Flag indicating whether the block has collision
Defaults to true
solid
:
Flag indicating whether the block gets flushed away with water.
Defaults to true
pushReaction
:
Specifies how the block reacts to being pushed by a piston. Possible values include normal, destroy, block
Defaults to normal
Block Behaviours
Block behaviours can be customized to add unique properties to blocks. All behaviours are optional, and some can be applied to both, blocks and decorations.
Blocks also work with most item-behaviours to give the blocks' item special features.
Some block behaviours provide blockstate properties you will have to provide models for, like axis
, repeater
, crop
, directional
, etc.
Example of a blockResource entry for the repeater
behaviour (can be found as relay block in the example datapack):
Click to expand
{
"blockResource": {
"models": {
"facing=up,powered=false": "minecraft:custom/block/arcanery/relay/relay_up_off",
"facing=down,powered=false": "minecraft:custom/block/arcanery/relay/relay_down_off",
"facing=north,powered=false": "minecraft:custom/block/arcanery/relay/relay_north_off",
"facing=south,powered=false": "minecraft:custom/block/arcanery/relay/relay_south_off",
"facing=east,powered=false": "minecraft:custom/block/arcanery/relay/relay_east_off",
"facing=west,powered=false": "minecraft:custom/block/arcanery/relay/relay_west_off",
"facing=up,powered=true": "minecraft:custom/block/arcanery/relay/relay_up_on",
"facing=down,powered=true": "minecraft:custom/block/arcanery/relay/relay_down_on",
"facing=north,powered=true": "minecraft:custom/block/arcanery/relay/relay_north_on",
"facing=south,powered=true": "minecraft:custom/block/arcanery/relay/relay_south_on",
"facing=east,powered=true": "minecraft:custom/block/arcanery/relay/relay_east_on",
"facing=west,powered=true": "minecraft:custom/block/arcanery/relay/relay_west_on"
}
}
}
Example of a block with behaviours set:
Click to expand
{
"id": "mynamespace:myblock",
"blockResource": {
"models": {
"...": "mynamespace:custom/block/myblock",
"...": "..."
}
},
"blockModelType": "full_block",
"properties": {
"destroyTime": 0,
"blockBase": "minecraft:stone",
"itemBase": "minecraft:paper"
},
"behaviour": {
"powersource": {
"value": 15
},
"repeater": {
"delay": 1,
"loss": 1
},
"fuel": {
"value": 10
},
"food": {
"hunger": 2,
"saturation": 1.0,
"canAlwaysEat": true,
"fastfood": true
},
"cosmetic": {
"slot": "chest",
"model": "mynamespace:custom/models/clown_backpack_animated",
"autoplay": "idle",
"scale": [1.5, 1.5, 1.5],
"translation": [0.0, 0.5, 0.0]
},
"strippable": {
"replacement": "minecraft:stone"
}
}
}
This creates a block + item that can be worn and when worn shows an animated blockbench model on the player. The item is also a food and can be used as fuel source in furnaces.
The block acts as a redstone powersource of level 15 and a repeater/relay and is strippable (turns to stone when stripped with an axe or an item with the stripper
item behaviour)
While possible, you probably don't want to combine powersource
with repeater
for actual blocks/items for obvious reasons.
Behaviours
axis
behaviour
Gives the block an axis
property/block-state similar to wooden logs/pillars and handles placement.
- Block-State-Properties to provide models for:
axis
: x, y, z
count
behaviour
Gives the block a count
property/block-state.
Works similar to turtle eggs or candles, allows you to place "multiple blocks/items" into one block.
- Block-State-Properties to provide models for:
count
: 1...max
facing
behaviour
Gives the block a facing
property/block-state similar to wooden logs/pillars and handles placement.
- Block-State-Properties to provide models for:
facing
: north, east, south, west, up, down
horizontal_facing
behaviour
Gives the block a facing
property/block-state similar to furnaces and handles placement.
Does not support up and down facing directions.
- Block-State-Properties to provide models for:
facing
: north, east, south, west
crop
behaviour
Makes the block behave like a crop, bonemeable, growing, minimum light requirement, etc. Also gives a growth bonus similar to vanilla crops, which check for farmland blocks in a 3x3 area centered below the crop block. The bonus block and radius can be configured.
You probably want to use this behaviour together with the can_survive
behaviour.
If you want your custom crop block to not turn farmland into dirt without moisture, like vanilla crops do, add your block to the block tag maintains_farmland
.
In order for the farmland to not turn into dirt when placing the crop on top of it, make sure the solid
property is set to false
For bee pollination, use the block tag bee_growables
.
You can make farmer villagers able to plant the seeds using the item tag villager_plantable_seeds
. Villagers will only work on crops that are on top of farmland blocks (vanilla limitation).
-
Block-State-Properties to provide models for:
age
: 0...maxAge-1
-
Fields:
maxAge
: maximum age steps of this block (from 0 to maxAge-1). Defaults to 4.minLightLevel
: Minimum light level this crop needs to survive. Defaults to 8.bonusRadius
: Radius to check for bonus blocks for. Defaults to 1.bonusBlock
: Bonus block to check for. More bonus blocks means faster growth. Defaults tominecraft:farmland
.villagerInteraction
: Allows farmer villagers to break and plant the custom crop. Defaults totrue
.beeInteraction
: Allows bees to pollinate the crop to increase its age. Defaults totrue
.
sapling
behaviour
Makes your block behave like vanilla saplings, growing based on random ticks and bonemealable.
All identifiers for the configured_placements are optional, they will only get used when configured.
You add your own configured placement for trees using vanilla datapack mechanics.
Checkout the example datapack for the test_tree block!
-
Block-State-Properties to provide models for:
stage
: 0 to 1. You can provide a single model to use for both states, usedefault
as key in that case.
-
Fields:
tree
: Identifier for a configured_placement (add via datapack or use vanilla ones)minLightLevel
: Defaults to9
secondaryChance
: Chance between 0 and 1 forsecondaryMegaTree
orsecondaryFlowers
placement to be used. Defaults to0
randomTickGrowthChance
: Defaults to0.15
bonemealGrowthChance
: Defaults to0.45
megaTree
: Identifier for a configured_placement. Will get used for 2x2 sapling placementssecondaryMegaTree
: Identifier for a configured_placement. Alternative tomegaTree
based onsecondaryChance
tree
: Identifier for a configured_placement. Normal tree without flowersecondaryTree
: Identifier for a configured_placement. Alternative totree
based onsecondaryChance
flowers
: Identifier for a configured_placement. Used when there is a flower neaby.secondaryFlowers
: Identifier for a configured_placement. Alternative toflowers
based onsecondaryChance
can_survive
behaviour
Checks for the block below with one of the configured block tags or blocks list. The block will break off, similar to flowers or crops, when the block below them is not supported.
The behaviour will automatically check for and apply any facing
or axis
block-state properties.
Useful for bushes/plants/crops/flowers and more
- Fields:
blocks
: List of blocks this block can survive on.- Example:
blocks: ["minecraft:stone", "minecraft:sand"]
- Example:
tags
: List of block-tags this block can survive on.- Example:
tags: ["minecraft:dirt", "minecraft:sculk_replaceable"]
- Example:
powersource
behaviour
Defines the block as a redstone power source.
Note: The field of this behaviour can be mapped to a block-state.
Example:
{
"behaviour": {
"powersource": {
"value": {
"age=0": 0,
"age=1": 15
}
}
}
}
Example with constant value:
{
"behaviour": {
"powersource": {
"value": 5
}
}
}
- Fields:
value
: The redstone power value the block emits (can be mapped). Defaults to 15
repeater
behaviour
Defines the block as a redstone repeater with configurable delay and loss.
-
Fields:
delay
: Delay in ticks. Defaults to 0loss
: Power loss during transfer. Defaults to 0
-
Block-State-Properties to provide models for:
powered
: true, falsefacing
: north, east, south, west, up, down
powerlevel
behaviour
Supplies a powerlevel
blockstate and changes to it depending on the input redstone signal.
-
Fields:
max
: Maximum powerlevel this block can display
-
Block-State-Properties to provide models for:
powerlevel
: 0...max-1
strippable
behaviour
Defines the block as strippable, replacing it with another block when interacted with an axe.
- Fields:
replacement
: The identifier of the block to replace the current block with. Example:minecraft:stone
lootTable
: Identifier for a loot table to use when the block is stripped. Example:minecraft:bell
slab
behaviour
Defines the block as slab, top, bottom, double, with placements, waterloggable.
- Block-State-Properties to provide models for:
type
: top, bottom, doublewaterlogged
: true, false
trapdoor
behaviour
Trapdoor like block.
-
Block-State-Properties to provide models for:
facing
: north, south, east, west, up, downhalf
: top, bottomopen
: true, falsewaterlogged
: true, false
-
Fields:
canOpenByWindCharge
: Whether the trapdoor can be opened by a wind charge. Defaults totrue
canOpenByHand
: Whether the trapdoor can be opened by hand. Defaults totrue
openSound
: Open sound. Defaults to wooden trapdoor open sound.closeSound
= Close sound. Defaults to wooden trapdoor close sound.
door
behaviour
Door-like "block" that is 2 blocks high. Comes with all door block state properties (hinge, open, powered, etc.)
-
Block-State-Properties to provide models for:
facing
: north, south, east, west, up, downhalf
: lower, upperopen
: true, falsehinge
: left, right
-
Fields:
canOpenByWindCharge
: Whether the door can be opened by a wind charge. Defaults totrue
canOpenByHand
: Whether the door can be opened by hand. Defaults totrue
openSound
: Open sound. Defaults to wooden door open sound.closeSound
= Close sound. Defaults to wooden door close sound.
simple_waterloggable
behaviour
Simple waterloggable block.
drop_xp
behaviour
Makes the block drop xp when being mined without the silk-touch enchantment.
The values of the min
and max
fields can be mapped to block-states.
Example:
{
"behaviour": {
"drop_xp": {
"min": {
"age=0": 0,
"age=1": 0,
"age=2": 4
},
"max": {
"age=0": 0,
"age=1": 0,
"age=2": 6
}
}
}
}
Example with constant values:
{
"behaviour": {
"drop_xp": 6
}
}
- Fields:
min
: Minimum amount of XP to dropmax
: Maximum amount of XP to drop
oxidizable
behaviour
Defines the block as oxidizing block, similar to the vanilla copper blocks, randomly replacing it with another block when it "ages". Can be reverted/scraped by axes and resets with lightning bolts like vanilla copper blocks.
- Fields:
replacement
: The identifier of the block to replace the current block with (e.g., "minecraft:stone").weatherState
: The current weathering state of this block. Can beunaffected
,exposed
,weathered
,oxidized
. Defaults tounaffected
. AweatherState
ofoxidized
will not oxidize any further.
budding
behaviour
With this behaviour the blocks grows other blocks, similar to budding amethyst blocks. The sides, blocks and chance can be configured.
If the blocks in grows
have directional/facing block state properties, they direction of the side the block is growing from will be set.
- Fields:
chance
: Chance of the block to grow another block or move a block to the next growth stage in percent, from 0 to 100. Defaults to 20sides
: List of sides blocks can grow out. Can benorth
,south
,east
,west
,up
ordown
. Defaults to all directionsgrows
: List of id's of blocks for the growth stages. Example:["minecraft:chain", "minecraft:end_rod"]
falling_block
behaviour
Makes the block a gravity affected/falling block like sand or anvils.
Note: All fields of this behaviour can be mapped to a block-state.
Fields:
delayAfterPlace
: Delay in ticks before the block falls. Defaults to 2heavy
: To cause anvil-like damage. Defaults to falsedamagePerDistance
: Accumulated damage per block fallenmaxDamage
: Maximum damage a falling block can dealdisableDrops
: Prevent the block from being placed when it fallssilent
: Flag whether sounds are played when the block falls or breakslandSound
: Sound played when the block landsbreakSound
: Sound played when the block breakscanBeDamaged
: Flag whether the block should be placed as the block indamagedBlock
damagedBlock
: New block to use when the falling block 'breaks'. Will copy applicable block state propertiesbaseBreakChance
: Chance for the block to break into the block indamagedBlock
on its ownbreakChancePerDistance
: Chance increase per block fallen for the block to break into the block indamagedBlock
Example:
{
"falling_block": {
"delayAfterPlace": 2, // delay in ticks before the block falls
"heavy": true, // to cause anvil-like damage
"damagePerDistance": 2.0, // accumulated damage per block fallen
"maxDamage": 40, // maximum damage
"disableDrops": false, // prevent the block from being placed
"silent": false, // no sounds
"landSound": "minecraft:block.anvil.land",
"breakSound": "minecraft:block.anvil.destroy",
"canBeDamaged": true, // flag wether the block should be placed as the block in "damagedBlock"
"damagedBlock": "minecraft:diamond_block", // new block to use, will copy applicable block state property
"baseBreakChance": 0.05, // chance for the block to "break" to the block in "damagedBlock"
"breakChancePerDistance": 0.05 // chance increase per block fallen
}
}
tnt
behaviour
With this behaviour the block can be lit with flint and steel or redstone to spawn a TNT entity with the blockstate of this block.
Note: All fields of this behaviour can be mapped to a block-state.
Fields:
- unstable: Flag whether the block explodes when a player tries to break it. Defaults to
false
- explosionPower: Explosion power. Defaults to
4.0
- fuseTime: Fuse time (delay until the tnt entity explodes). Defaults to
80
- primeSound: Sound to play when the block is primed. Defaults to
minecraft:entity.tnt.primed
Block Examples
See the datapack in the GitHub repository for examples!
https://github.com/tomalbrc/filament/tree/1.21/example_datapack
Note: All fields support either camelCase or snake_case!
Decorations
File
Decoration configuration files are to be placed in MyDatapack/data/<namespace>/filament/decoration/my_decoration.json
.
All item-behaviours such as food
, fuel
and cosmetic
are supported by decorations.
You can also set components similar to item configurations using the components
field
Contents
{
"id": "mynamespace:clown_horn",
"vanillaItem": "minecraft:paper",
"itemResource": {
"models": {
"default": "mynamespace:custom/misc/clown_horn"
}
},
"group": "mynamespace:mygroup",
"properties": {
"stackSize": 1
},
"behaviour": {
"instrument": {
"sound": "mynamespace:misc.honk",
"range": 64,
"useDuration": 60
}
},
"components": {
// ...
}
}
The file contents are very similar to that of blocks, except for additional behaviours exclusive to decorations.
Decorations do not support most of the block behaviours.
Properties
Decoration properties share the same properties as items and a few of blocks, like solid
and pushReaction
Example of properties:
{
"properties": {
"rotate": false,
"rotateSmooth": false,
"placement": {
"floor": true,
"wall": false,
"ceiling": false
},
"glow": false,
"waterloggable": true,
"solid": false,
"display": "fixed",
"blockBase": "minecraft:stone",
"useItemParticles": true,
"showBreakParticles": true
}
}
rotate
:
Boolean (true/false) indicating whether the decoration can rotate (90° intervals)
Defaults to false
rotateSmooth
:
Boolean (true/false) indicating whether the decoration can rotate in 45° intervals
Defaults to false
placement
:
A set of options for placement options. Possible keys: "floor", "wall", "ceiling".
Default values:
{
"placement": {
"floor": true,
"wall": false,
"ceiling": false
}
}
glow
:
Boolean (true/false) indicating whether the decoration ignores ambient light and assumes light level 15.
Defaults to false
waterloggable
:
Boolean (true/false) indicating whether the decoration blocks can be waterlogged.
Defaults to true
solid
:
Boolean (true/false) indicating whether the decoration is solid - can be flushed away by water if set to false.
Defaults to true
display
:
Changes the item_display value used for the Item Display Entity of the decoration.
Can be none
, thirdperson_lefthand
, thirdperson_righthand
, firstperson_lefthand
, firstperson_righthand
, head
, gui
, ground
, and fixed
.
Defaults to fixed
.
showBreakParticles
Flag whether to show break particles.
Defaults to true
blockBase
Used for place & break sounds and particles if useItemParticles
is set to false
Defaults to minecraft:stone
useItemParticles
Flag whether to use item particles. Uses the particles of the block defined in blockBase
if set to false
.
Defaults to true
showBreakParticles
Flag whether to show break particles at all.
Defaults to true
Decoration Behaviours
Example of some behaviours for decorations:
{
"id": "mynamespace:clown_horn",
"vanillaItem": "minecraft:paper",
"itemResource": {
"models": {
"default": "mynamespace:custom/misc/clown_horn"
}
},
"behaviour": {
"animation": {
"model": "mynamespace:mymodel",
"autoplay": "myAnimationName"
},
"container": {
"name": "Example Container",
"size": 9,
"purge": false,
"openAnimation": "openAnimation",
"closeAnimation": "closeAnimation"
},
"lock": {
"key": "minecraft:tripwire_hook",
"consumeKey": false,
"discard": false,
"unlockAnimation": "unlockAnimation",
"command": "say Unlocked"
},
"seat": [
{
"offset": [0.0, 0.0, 0.0],
"direction": 0.0
}
],
"showcase": [
{
"offset": [0.0, 0.0, 0.0],
"scale": [1.0, 1.0, 1.0],
"rotation": [0.0, 0.0, 0.0, 1.0],
"type": "item",
"filterItems": null,
"filterTags": null
}
],
"cosmetic": {
"slot": "head",
"model": "mynamespace:clown_backpack_animated",
"autoplay": "idle",
"scale": [1.5, 1.5, 1.5],
"translation": [0.0, 0.5, 0.0]
}
}
}
animation
behaviour
Defines an animation behaviour for decorations. Supports ajmodel/bbmodels.
Models are placed in data/mynamespace/filament/model/mymodel.bbmodel
.
You would reference it as mynamespace:mymodel
in the model
field.
Fields:
model
: The name of the animated model associated with this animation (if applicable).autoplay
: The name of the animation to autoplay (if specified).
Example:
{
"animation": {
"model": "mynamespace:mymodel",
"autoplay": "myAnimationName"
}
}
container
behaviour
Defines a container behaviour for decorations.
Dropper/Hopper support is not implemented yet as of filament 0.10.7
Allows to create chests, trashcans, etc.
Works with the animation
behaviour to play an animation defined in the bbmodel/ajmodel.
Fields:
name
: The name displayed in the container UI.size
: The size of the container, has to be 5 slots or a multiple of 9, up to 6 rows of 9 slots.purge
: Indicates whether the container's contents should be cleared when no player is viewing the inventory.openAnimation
: The name of the animation to play when the container is opened (if applicable).closeAnimation
: The name of the animation to play when the container is closed (if applicable).
Example:
{
"container": {
"name": "Example Container",
"size": 9,
"purge": false,
"openAnimation": "openAnimation",
"closeAnimation": "closeAnimation"
}
}
lamp
behaviour
Allows you to create lamps that either switch on/off or cycle through a list of light levels on player interaction.
Fields:
on
: Light level to use for the 'on' stateoff
: Light level to use for the 'off' statecycle
: List of light levels to cycle through.
On/off lamp example:
{
"lamp": {
"on": 15,
"off": 0
}
}
Cycling lamp example:
{
"lamp": {
"cycle": [0, 2, 4, 6, 8, 10, 12, 14]
}
}
interact_execute
/ lock
behaviour
Defines a behaviour that runs a command, for decorations.
This behaviour is available under 2 names, interact_execute
and lock
. The name lock
exists to keep compatibility with older versions of filament / filament configs.
It's an analog to the execute
item behaviour.
The command will only run once if a key is specified
Fields:
key
: The identifier of the key required to unlock.consumeKey
: Determines whether the key should be consumed upon unlocking.discard
: Specifies whether the lock utility should be discarded after unlocking.unlockAnimation
: Name of the animation to play upon successful unlocking (if applicable).command
: Command to execute when the lock is successfully unlocked (if specified).
seat
behaviour
Defines a seating behaviour for decorations.
For chairs, benches, etc.
Fields:
offset
: The player seating offset.direction
: The rotation offset of the seat in degrees. Defaults to180
Single seat example:
{
"seat": [
{
"offset": [0.0, 0.0, 0.0],
"direction": 0.0
}
]
}
showcase
behaviour
Defines a showcase behaviour for decorations.
Allows you to create shelves / item-frame like decorations.
Fields:
offset
: Offset for positioning the showcased item.scale
: Scale of the showcased item.rotation
: Rotation of the showcased item.type
: Type to display, block for blocks (block display), item for items (item display), dynamic uses blocks if possible, otherwise item (block/item display).filterItems
: Items to allow.filterTags
: Items with given item tags to allow.
Single item showcase example:
{
"showcase": [
{
"offset": [0.0, 0.0, 0.0],
"scale": [1.0, 1.0, 1.0],
"rotation": [0.0, 0.0, 0.0, 1.0],
"type": "item",
"filterItems": ["minecraft:paper"],
"filterTags": ["minecraft:tag_example"]
}
]
}
cosmetic
behaviour
Defines cosmetic behaviours for decorations, supporting animated Blockbench models for chestplates and simple item models.
Cosmetics are worn on the player using item display entities (except for the head slot)
Fields:
slot
: The equipment slot for the cosmetic (head or chest).model
: Optional, the resource location of the animated blockbench or animated-java model for the cosmetic.autoplay
: Optional, the name of the animation to autoplay, which should be loopable.scale
: Scale of the chest cosmetic. Defaults to[1 1 1]
translation
: Translation of the chest cosmetic. Defaults to[0 0 0]
.
Backpack example:
{
"cosmetic": {
"slot": "chest",
"model": "mynamespace:clown_backpack_animated",
"autoplay": "idle",
"scale": [1.5, 1.5, 1.5],
"translation": [0.0, 0.5, 0.0]
}
}
Blocks
The blocks
field allows to specify where barriers blocks should be placed for the decoration, instead of client-side interaction entities.
It also allows for gaps:
{
"blocks": [
{
"origin": [0.0, 0.0, 0.0],
"size": [1.0, 1.0, 1.0]
},
{
"origin": [2.0, 2.0, 2.0],
"size": [1.0, 1.0, 1.0]
}
// Add more block config objects as needed
]
}
2 block high block configuration:
{
"blocks": [
{
"origin": [0.0, 0.0, 0.0],
"size": [1.0, 2.0, 1.0]
}
]
}
Simple and complex decorations
All decorations with behaviours, dye-able vanilla items or with a blocks
configuration larger that a single block, are considered to be "complex".
The difference between simple and complex decorations gameplay wise is that complex decorations are not pushable by pistons.
When it comes to the implementation, complex decorations use block entities to store data, while simple decorations are made up of a single, "simple", block.
Decoration Examples
Note: All fields support either camelCase or snake_case!
Animated chest with solid 1x1 solid collision:
{
"id": "mynamespace:example_chest",
"itemResource": {
"models": {
"default": "mynamespace:custom/furniture/chests/example_chest"
}
},
"properties": {
"rotate": true, // to allow 90° rotations
"rotateSmooth": true // to allow 45° rotations
},
"behaviour": {
"animation": {
"model": "my_filament_namespace:example_chest"
},
"container": {
"name": "Example Chest",
"size": 36,
"purge": false,
"openAnimation": "open",
"closeAnimation": "close"
}
},
"blocks": [
{
"origin": [0,0,0],
"size": [1,1,1]
}
]
}
YAML:
id: mynamespace:example_chest
item_resource:
models:
default: mynamespace:custom/furniture/chests/example_chest
properties:
rotate: true
rotate_smooth: true
behaviour:
animation:
model: my_filament_namespace:example_chest
container:
name: Example Chest
size: 36
purge: false
open_animation: open
close_animation: close
blocks:
- origin: [0, 0, 0]
size: [1, 1, 1]
The animation
behaviour gets used for animations by various behaviours such as the container
behaviour.
The blockbench model gets loaded from a filament datapack that contains the referenced model using the provided namespace
The lock
behaviour also supports an animation using the animation
behaviour
Beach umbrella with custom size:
{
"id": "mynamespace:beach_umbrella_top",
"itemResource": {
"models": {
"default": "mynamespace:custom/furniture/umbrella/beach_umbrella_top"
}
},
"vanillaItem": "minecraft:leather_horse_armor",
"properties": {
"rotate": true,
"rotateSmooth": true
},
"size": [3, -0.5]
}
The size
field creates an interaction entity with a custom size instead of the default 1x1 sized one when no blocks
are set.
Pile of gold ingots with solid 1x1 solid collision:
{
"id": "mynamespace:pile_of_gold_ingots",
"model": "mynamespace:custom/deco/misc/pile_of_gold_ingots",
"itemResource": {
"models": {
"default": "mynamespace:custom/deco/misc/pile_of_gold_ingots"
}
},
"properties": {
"rotate": true,
"rotateSmooth": true
},
"blocks": [
{
"origin": [0,0,0],
"size": [1,1,1]
}
]
}
TODO: Write some tutorials (with pictures)
Creating armor
TODO for <=1.21.1 and >=1.21.2:
- Texture
- Where to save it in the resourcepack
- Creating the config
- Enabling autohost
Creating a cosmetic
TODO:
- Blockbench model / texture, how to align chest and hat stuff
- Where to save both in the resourcepack
- Creating the config
- Enabling autohost
Creating a simple block
TODO:
- Blockbench model
- Where to save in the resourcepack
- Creating the config
- Enabling autohost
Creating a simple item
TODO:
- Blockbench model / texture
- Where to save both in the resourcepack
- Creating the config
- Enabling autohost
Creating a sword
TODO:
- Blockbench model / texture, how to align chest and hat stuff
- Where to save both in the resourcepack
- Creating the config
- datapack / item tags
- Enabling autohost
Adding a custom tree / sapling
TODO:
- Blockbench model / texture
- Where to save both in the resourcepack
- Creating the config
- datapack / configured_placement
- Enabling autohost